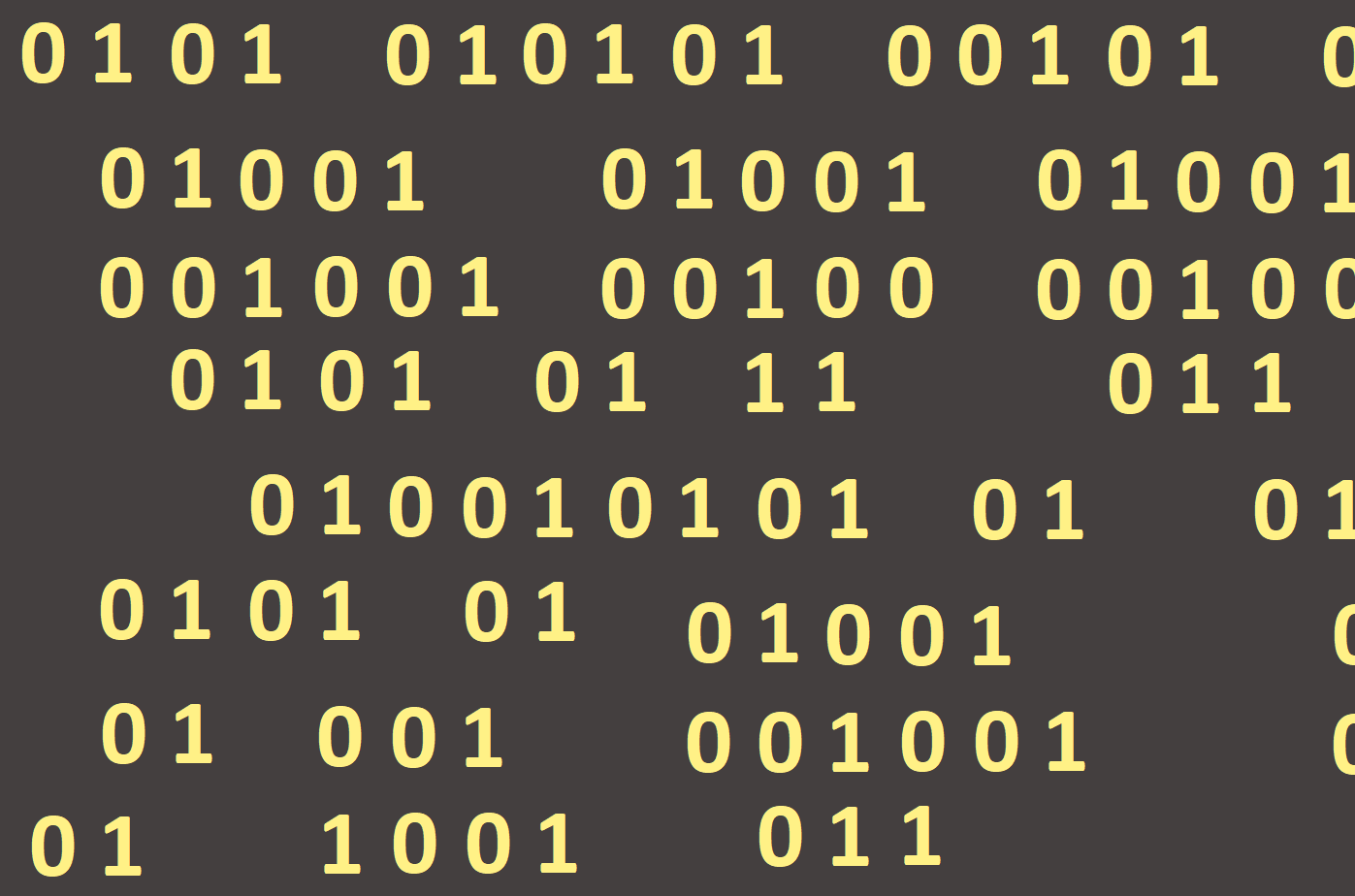
Character strings
The character string is a type of data very similar to a array. It contains any sequence of characters writable from the keyboard. The maximum length of the text string is 255 characters.
It is marked with a string keyword. There is no universal chain type introduced which would include chains of any length, but their length distinguishes different types of strings. Above we have said that the string is a sequence of characters. The variable of this type can remember one character only or a maximum length of 255 characters, e. g. a letter (A, B, C,...a, b, c,...), a digit (0, 1, 2,...) but also other characters (&, @, +, -, *, /,...) with a char value occupying one byte on the computer and coded with an ASCII encoding.
The operations we can perform with character strings are for example an assignment of a value in the variable, we can compare it to a different value, and we can write out the character value into the graphical area.
Key features with character strings are:
- Chain creation
- Chain length detection
- Chaining of two or more strings together
- Work with individual chain characters
- Calculation of characters in the specified string
- Chain copying
The character strings (String), as well as the char type, have their own constants that we write into apostrophes. We have already encountered with these constants, for example when ordering a text listing command TextOut (Image1.Canvas.TextOut(10, 10, 'Hello')), or when you set a Label component text (Label1.Caption := 'Hello').
In the following examples, we will show how we can keep these texts in variables, how we can change them and how we can work with them. First of all, we must have declared character string variables (String), then assign them some value, e. g. chain constant, and finally, in our already known way, we will write it out on the graphical area (Figure 83).
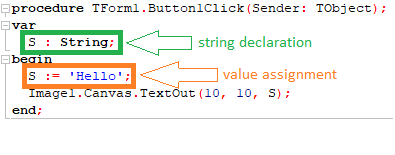
Once we have the chain created, we can use the Length function to find out how many characters are included in the string, but it is of the Integer type and so we have to declare a new Integer type variable (Figure 84).
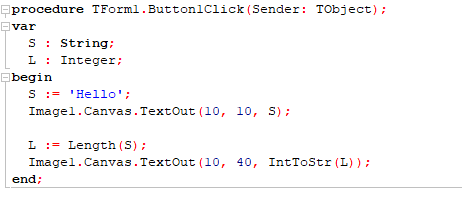
After running the program (F9) we see following (Figure 85):
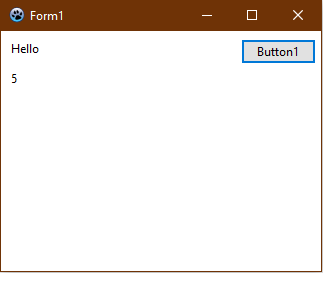
The program told us that the String "Welcome!" has a length 8 (it consists of 8 characters). We will change the program so that it will not only write out the number eight, but will answer us with the whole sentence (Figure 86) "I found out the length of the string. Its length is 8" (Figure 87).
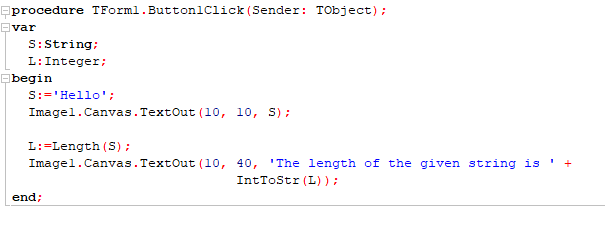
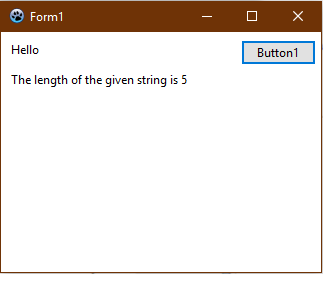
The function that allows us to combine two or more strings into one set is called concatenation and uses the plus (+) operator. In this case, it has a different meaning than when numbering.
The string variable can also contain an empty string (String := '') and its length is 0.
We can also work with a single string character. If we have a string variable, we can access a specific character in a way that the index, that is a serial number of the character, will be closed in square brackets (ALT + 91 and ALT + 93). Characters in the string are numbered from 1 for the first character to the Length for the last character.
Let´s work with our created "Welcome!" chain which has a length of 8, as we have already found out in the previous example, therefore the index can be a number from 1 to 8. For example:
R [4] has the value 'a'
R [Length (R)] is the last character in the string, that is '!'
R [I] is the I-character, dependent on the value of the Integer variable, which must be
from the interval <1, 8>
The entered index can not only be an Integer constant, but also a variable, or an arithmetic expression. It is important that it has a value from the interval <1, Length (R)>.
We will show the work with individual string characters in the following example, in which we will also use a For loop and the Edit1 component. The program will sequentially select a character from the specified string of characters, sets the random font colour each time and prints the corresponding character (Figure 88).
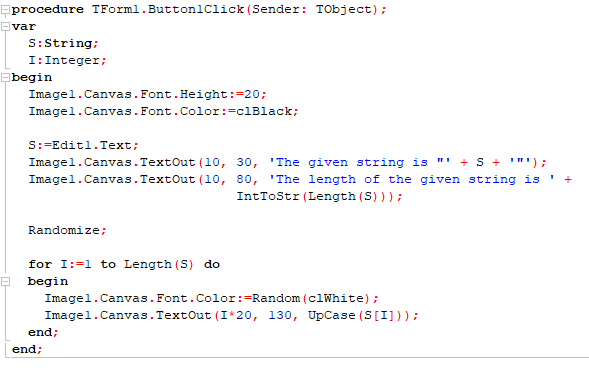
- Before we worked with the string characters, we set the colour and the font size that remains unchanged. If we do not specify these commands, the program would also change the colour of first two lines, which we do not want.
- So far, we have only worked with apostrophes if we have defined some text. In this example, we have defined apostrophes, which is why the quoted text is written in apostrophes.
- We have just used one new command, an UpCase feature that transforms a lower case letter to the capital letter. Other characters (e. g. %, &, @,...) remain unchanged.
- Note! In the Object Inspector, we have set the Text attribute of the Edit component to a zero value.
When we run the program, we enter a text (such as Welcome!) in the Edit component with which the program will work and it will be edited. It may look like this (Figure 89):
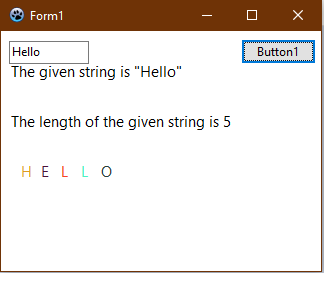
The following program calculates the number of letters, digits, and blank spaces in the given text. In the For loop we pass through all the characters and for each individually (R[I]) we find whether it is a letter, a digit or a blank space (Figure 90).
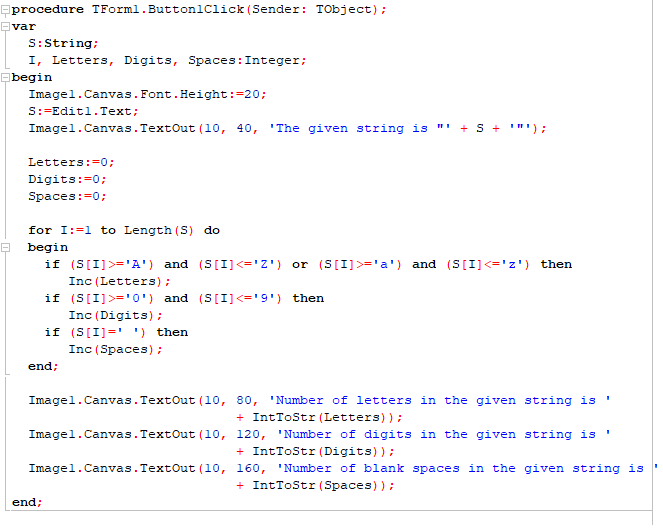
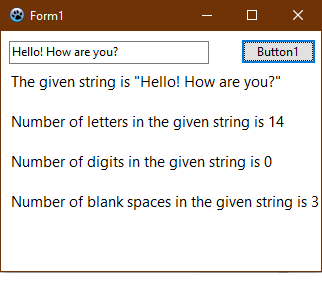
We have used several new commands, which we will now explain:
In the conditional command, we entered logical operations and and or. Except for them, the operation not exists as well:
- not A - negation - True, if A = False
- A and B - logical conjunction - True, if A = True and also B = True
- A or B - logical disjunction - True, if at least one A = True or B = True
Another new command was an Inc command with which we can increase the ordinal content of the variable (distinguishing between the preceding and the following element only in the sense of "greater - smaller"). Other features include:
- Succ ('A') = 'B' - successor of the ordinal value
- Succ ('f') = 'g' - successor of the ordinal value
- Pred ('3') = '2' - predecessor of the ordinal value
- Pred ('Z') = 'Y' - predecessor of the ordinal value
- Inc (I) equal to I := I +1; - increasing of the ordinal value
- Dec (Z) same as Z := Before (Z); - decreasing of the ordinal value
- Inc (X, 18) same as X := X +18; - increasing of the ordinal value
One of the most important features when working
with a text string is a Copy feature, which is able to select a sub-string out
of a string. This means that we do not have to select only one of the R string
character R [I] and continue to work with it, but we can also select a
contiguous part of it. The Copy feature has three parameters:
- It is a string itself from which we want to
select a part.
- The sub-string begins on this index.
- It is the length of this sub-string.
Copy ('Janosik', 3, 2) = 'no'
Often, we can meet with the following record
Copy (String, I, MaxInt)
which indicates that from the given chain String we take all the characters from the I-character to the end. For example, if we want to get the character on the I-th position, we can write:
Copy (Retazec, 1, I - 1) + Copy (Retazec, I + 1, MaxInt)
The resulting string is thus a combination of two strings: characters from the beginning to the character before the I-th and the chain from the next after the I-th to the end. Likewise, we will write if we want I-th character (only one character) to replace multiple, e. g. string ´...´.
Copy (Retazec, 1, I - 1 ) + '...' + Copy (Retazec, I + 1, MaxInt)
The following program lists the text several times: first only the first character, then the first two, the first three, ... to print the whole chain (Figure 92):
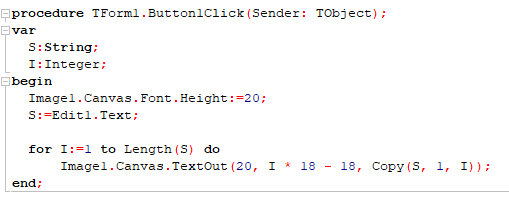
The program will list (Figure 93):
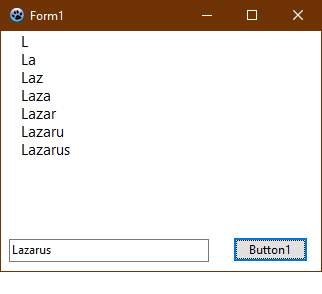
Other string functions include:
- UpperCase - writes a string in which all the letters are upper case
- LowerCase - writes a string in which all the letters are lower case
- Trim - writes a string without spaces at the beginning and the end
- Insert - inserts a character into the string
- Pos - looks for the position of the specific character/characters
- You can find additional string functions in the Free Pascal Help.